C# Dependency Injection
C# Dependency Injection is the topic of this blog article. Enjoy with the magic of learning.
Introduction
Dependency Injection (DI) is a fundamental technique in modern software development, promoting flexibility, maintainability, and testability. By understanding and implementing DI, developers can create applications that are easier to manage and scale. This article delves into the core concepts of DI, its benefits, and how it is utilized in C#.
What is Dependency Injection?
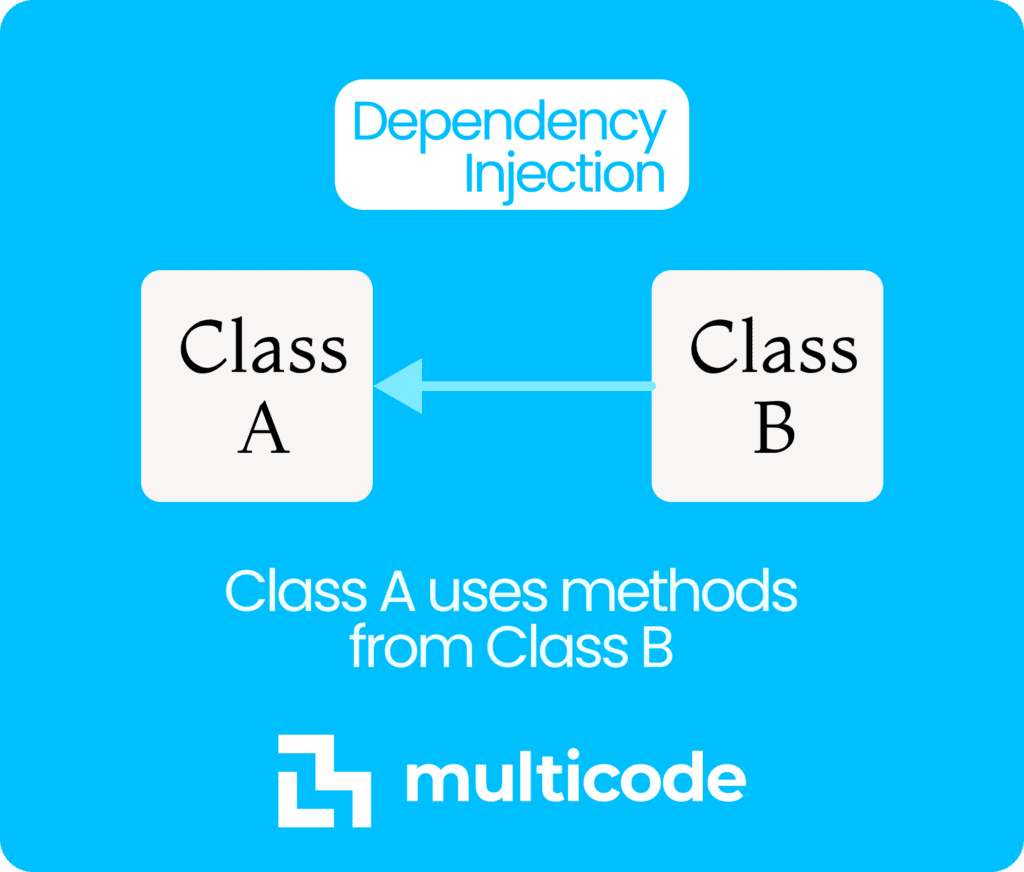
Dependency Injection is a design pattern that enables the decoupling of class dependencies, allowing objects to be supplied with their dependencies from an external source rather than creating them internally. This approach facilitates the inversion of control (IoC), where the control of creating and managing dependencies is transferred from the class to an external component or framework.
Dependency Injection in C#
In C# Dependency Injection can be implemented in various ways, including constructor injection, property injection, and method injection. Constructor injection is the most common method, where dependencies are provided through the class constructor. Here’s an example:
public class MyService
{
private readonly IMyDependency _myDependency;
public MyService(IMyDependency myDependency)
{
_myDependency = myDependency;
}
public void PerformAction()
{
_myDependency.Execute();
}
}
ASP.NET Core Dependency Injection
ASP.NET Core has built-in support for Dependency Injection, making it easy to configure and manage dependencies. For more details on how to implement Dependency Injection in ASP.NET Core, please visit ASP.NET Core Dependency Injection.
Autofac Dependency Injection
Autofac is a popular third-party Dependency Injection container for .NET. It provides advanced features and flexibility compared to the built-in DI container. To use Autofac in an ASP.NET Core application, you need to install the Autofac.Extensions.DependencyInjection package and configure it in the Program.cs
file:
var builder = WebApplication.CreateBuilder(args);
builder.Host.UseServiceProviderFactory(new AutofacServiceProviderFactory());
builder.Services.AddControllersWithViews();
builder.Services.AddRazorPages();
builder.Host.ConfigureContainer<ContainerBuilder>(containerBuilder =>
{
containerBuilder.RegisterType<MyDependency>().As<IMyDependency>();
});
var app = builder.Build();
Inversion of Control (IoC)
Inversion of Control (IoC) is a principle where the control of creating and managing dependencies is inverted from the class to an external component. Dependency Injection is one of the techniques to achieve IoC. This principle helps in creating loosely coupled and easily testable code. IoC containers like the built-in ASP.NET Core DI container or Autofac manage the lifecycle and resolution of dependencies.
Service Lifetimes in Dependency Injection
Proper management of service lifetimes is crucial for application performance and memory management. ASP.NET Core supports three primary lifetimes:
- Transient: Created each time they are requested. Useful for lightweight, stateless services.
- Scoped: Created once per request. Ideal for services that need to maintain state within a single request.
- Singleton: Created once and shared throughout the application’s lifetime. Suitable for services that maintain global state.
Understanding these lifetimes helps in making informed decisions about how services should be instantiated and managed within the application.
Constructor Injection in C#
Constructor injection is the most common and recommended method for injecting dependencies. It makes the dependencies explicit and ensures that the class cannot be instantiated without providing the required dependencies. Here’s an example:
public class UserService
{
private readonly IUserRepository _userRepository;
public UserService(IUserRepository userRepository)
{
_userRepository = userRepository;
}
public void CreateUser(User user)
{
_userRepository.Add(user);
}
}
Advanced Dependency Injection Techniques
Conditional Registration
Sometimes, you may need to register services conditionally based on certain criteria. This can be achieved using conditional logic during service registration:
if (someCondition)
{
builder.Services.AddSingleton<IService, ServiceImplementationA>();
}
else
{
builder.Services.AddSingleton<IService, ServiceImplementationB>();
}
Keyed Services
Keyed services allow for multiple implementations of the same interface, distinguishable by a key. This is useful when different implementations are needed based on a context or condition:
builder.Services.AddKeyedSingleton<ICache, BigCache>("big");
builder.Services.AddKeyedSingleton<ICache, SmallCache>("small");
Scoped Services in Middleware
Injecting scoped services into middleware can be done using the Invoke
or InvokeAsync
methods. This allows the middleware to have scoped dependencies that are resolved per request:
public async Task InvokeAsync(HttpContext context, IMyScopedService scopedService)
{
scopedService.DoWork();
await _next(context);
}
Best Practices for Dependency Injection
Avoid Over-Injection
If a class has too many dependencies, it might be a sign that it has too many responsibilities. Refactor the class to adhere to the Single Responsibility Principle.
Prefer Constructor Injection
Constructor injection makes dependencies explicit and the code easier to understand and maintain.
Design for Interface
Depend on abstractions (interfaces) rather than concrete implementations to promote flexibility and testability.
Dispose Services Properly
Ensure that services implementing IDisposable
are properly disposed of by the DI container. This is crucial for managing resources and preventing memory leaks.
Conclusion
Dependency Injection is a powerful design pattern that brings numerous benefits to software development. By leveraging C# Dependency Injection, developers can create applications that are more modular, testable, and maintainable. Understanding the core concepts and best practices of DI will help in building robust and scalable software solutions.
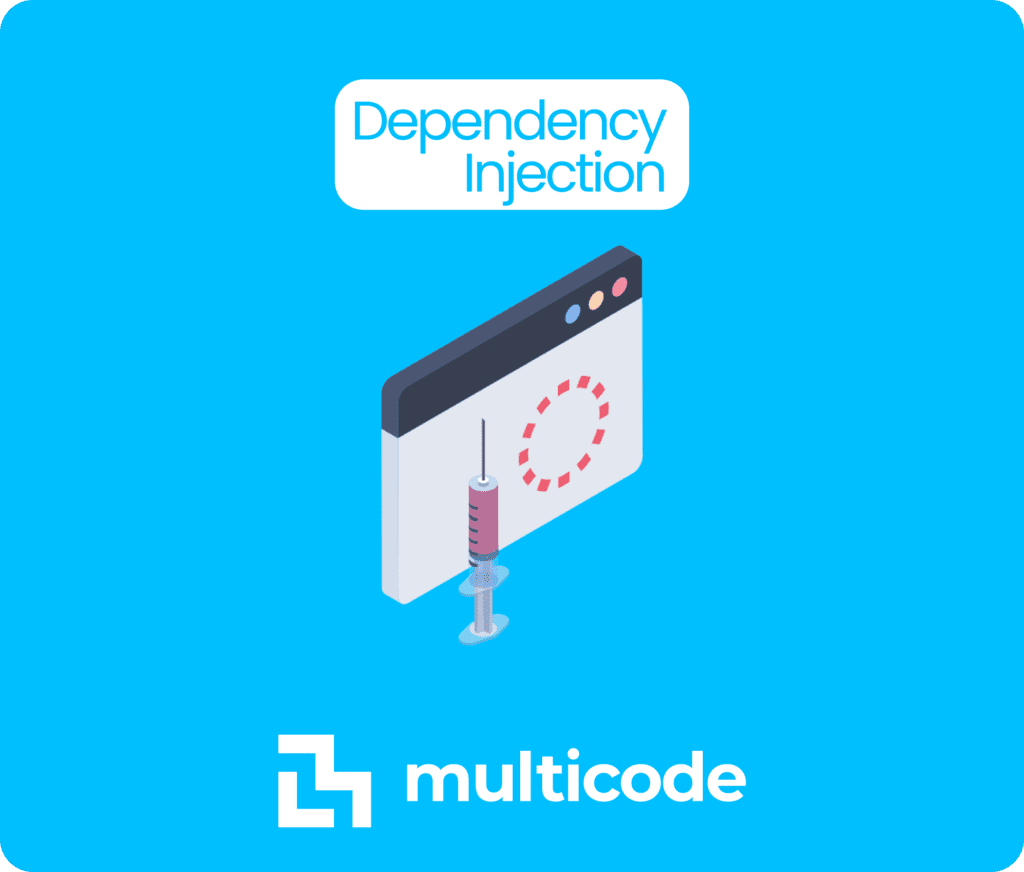
An example of C# Dependency Injection shows how classes receive their dependencies from the outside. For instance, the MyService
class has a dependency on IMyDependency
, which is provided through the constructor.
Autofac is a popular third-party Dependency Injection container for .NET. It provides advanced features and flexibility compared to the built-in DI container. To use Autofac in an ASP.NET Core application, you need to install the Autofac.Extensions.DependencyInjection package and configure it in the Program.cs
file.
C# Console App Dependency Injection involves registering services in the DI container and then resolving them in the console application. This allows the console app to utilize DI just like other types of applications.
C# Class Library Dependency Injection refers to applying dependency injection within class libraries. This enables classes in the library to receive their dependencies from the outside, making them more flexible and testable.
C# Static Class Dependency Injection can be challenging because static classes do not support constructor injection. However, alternative methods such as providing dependencies through static properties can be used.
C# Unit Test Dependency Injection involves using dependency injection in unit tests. This allows the dependencies of the class being tested to be replaced with mock objects or special test dependencies.
C# Web API Dependency Injection refers to applying dependency injection in web API projects. This enables API controllers and services to receive their dependencies through the DI container.
A C# Dependency Injection Framework is a framework that supports dependency injection. Frameworks like ASP.NET Core and Autofac manage dependencies and make different parts of the application more flexible and testable.
A C# Dependency Injection Container is a structure that registers and resolves dependencies. The built-in DI container in ASP.NET Core or third-party containers like Autofac can be used to manage dependencies.
C# Minimal API Dependency Injection refers to applying dependency injection in minimal API projects. This allows minimal API configurations and services to be managed through the DI container.
Results
#1. What is Dependency Injection (DI)?
#2. Which method registers services with Dependency Injection in ASP.NET Core?
#3. What is Autofac?
#4. What is Constructor Injection?
#5. Which service lifetime creates a service once per request?
#6. How is C# Console App Dependency Injection implemented?
#7. What is C# Class Library Dependency Injection?
#8. Is C# Static Class Dependency Injection possible?
#9. How is C# Unit Test Dependency Injection used?
#10. What is C# Web API Dependency Injection?
Internal and External Linking
For further reading on Dependency Injection, you can explore the following resources:
- Dependency Injection in ASP.NET Core
- C# Dependency Injection