ASP.NET Core Dependency Injection
Introduction
Dependency Injection (DI) is a fundamental technique in modern software development, promoting flexibility, maintainability, and testability. By understanding and implementing DI, developers can create applications that are easier to manage and scale. This article delves into the core concepts of DI, its benefits, and how it is utilized in ASP.NET Core.
What is Dependency Injection?
Dependency Injection is a design pattern that enables the decoupling of class dependencies, allowing objects to be supplied with their dependencies from an external source rather than creating them internally. This approach facilitates the inversion of control (IoC), where the control of creating and managing dependencies is transferred from the class to an external component or framework.
Core Concepts of Dependency Injection
- Service Registration: Dependencies are registered in a service container, typically in the
Startup.cs
orProgram.cs
file. These services can have different lifetimes:- Transient: Created each time they are requested.
- Scoped: Created once per request.
- Singleton: Created once and shared throughout the application’s lifetime.
- Service Resolution: The DI container resolves dependencies by injecting them into constructors of the classes where they are needed.
- Service Lifetimes: Proper management of service lifetimes is crucial for application performance and memory management. ASP.NET Core supports three primary lifetimes:
- Transient: Useful for lightweight, stateless services.
- Scoped: Ideal for services that need to maintain state within a single request.
- Singleton: Suitable for services that maintain global state.
Benefits of Dependency Injection
- Decoupling: By injecting dependencies, classes are decoupled from the concrete implementations, allowing for easier substitution of components.
- Testability: DI facilitates unit testing by enabling the use of mock dependencies.
- Maintainability: Changes in dependencies do not require changes in the classes that use them, reducing the impact of code changes.
- Flexibility: DI allows for easy configuration and reconfiguration of dependencies without modifying the classes that use them.
Implementing Dependency Injection in ASP.NET Core
Registering Services
In ASP.NET Core, services are registered in the Program.cs
file. Here’s an example:
var builder = WebApplication.CreateBuilder(args);
builder.Services.AddTransient<IMyDependency, MyDependency>();
builder.Services.AddScoped<IOtherDependency, OtherDependency>();
builder.Services.AddSingleton<IAnotherDependency, AnotherDependency>();
var app = builder.Build();
Injecting Services
Once registered, services can be injected into controllers or other classes using constructor injection:
public class MyController : Controller
{
private readonly IMyDependency _myDependency;
public MyController(IMyDependency myDependency)
{
_myDependency = myDependency;
}
public IActionResult Index()
{
_myDependency.DoWork();
return View();
}
}
Advanced Dependency Injection Techniques
Conditional Registration
Sometimes, you may need to register services conditionally based on certain criteria:
if (someCondition)
{
builder.Services.AddSingleton<IService, ServiceImplementationA>();
}
else
{
builder.Services.AddSingleton<IService, ServiceImplementationB>();
}
Keyed Services
Keyed services allow for multiple implementations of the same interface, distinguishable by a key:
builder.Services.AddKeyedSingleton<ICache, BigCache>("big");
builder.Services.AddKeyedSingleton<ICache, SmallCache>("small");
Scoped Services in Middleware
Injecting scoped services into middleware can be done using the Invoke
or InvokeAsync
methods:
public async Task InvokeAsync(HttpContext context, IMyScopedService scopedService)
{
scopedService.DoWork();
await _next(context);
}
Best Practices for Dependency Injection
- Avoid Over-Injection: If a class has too many dependencies, it might be a sign that it has too many responsibilities. Refactor to adhere to the Single Responsibility Principle.
- Prefer Constructor Injection: It makes dependencies explicit and the code easier to understand and maintain.
- Design for Interface: Depend on abstractions (interfaces) rather than concrete implementations to promote flexibility and testability.
- Dispose Services Properly: Ensure that services implementing
IDisposable
are properly disposed of by the DI container.
Conclusion
Dependency Injection is a powerful design pattern that brings numerous benefits to software development. By leveraging DI in ASP.NET Core, developers can create applications that are more modular, testable, and maintainable. Understanding the core concepts and best practices of DI will help in building robust and scalable software solutions.
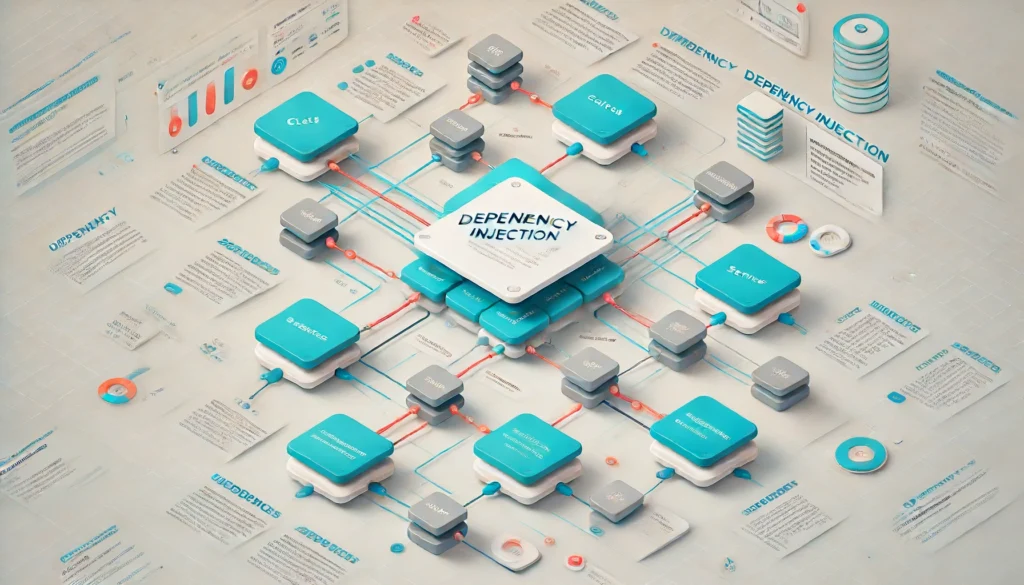
1. What is Dependency Injection?
Dependency Injection (DI) is a design pattern that allows the decoupling of class dependencies. It enables objects to be supplied with their dependencies from an external source, rather than creating them internally, facilitating the inversion of control (IoC).
2. Why is Dependency Injection important in modern software development?
Dependency Injection promotes flexibility, maintainability, and testability. It helps create applications that are easier to manage and scale by decoupling classes from their dependencies, allowing for easier substitution of components, and enabling effective unit testing.
3. What are the core concepts of Dependency Injection?
The core concepts of DI include:
Service Registration: Registering dependencies in a service container.
Service Resolution: Resolving dependencies by injecting them into constructors.
Service Lifetimes: Managing service lifetimes (Transient, Scoped, Singleton) for performance and memory management.
4. How are services registered in ASP.NET Core?
In ASP.NET Core, services are registered in the Program.cs
file using methods like AddTransient
, AddScoped
, and AddSingleton
.
Example:
var builder = WebApplication.CreateBuilder(args);
builder.Services.AddTransient();
builder.Services.AddScoped();
builder.Services.AddSingleton();
var app = builder.Build();
5.How are services injected in ASP.NET Core?
Once registered, services are injected into controllers or other classes using constructor injection.
Example down below
6. What are the different service lifetimes supported by ASP.NET Core?
ASP.NET Core supports three primary service lifetimes:
Transient: Created each time they are requested. Useful for lightweight, stateless services.
Scoped: Created once per request. Ideal for services that need to maintain state within a single request.
Singleton: Created once and shared throughout the application’s lifetime. Suitable for services that maintain global state.
7. What are the benefits of using Dependency Injection?
Benefits include:
– Decoupling: Classes are decoupled from concrete implementations.
– Testability: Enables effective unit testing by allowing the use of mock dependencies.
– Maintainability: Changes in dependencies do not require changes in dependent classes.
– Flexibility: Easy configuration and reconfiguration of dependencies without modifying dependent classes.
8. How can services be registered conditionally in ASP.NET Core?
Services can be registered conditionally based on certain criteria.
Example:
9. How are scoped services injected into middleware in ASP.NET Core?
Scoped services can be injected into middleware using the Invoke or InvokeAsync methods.
Example:
10. What are some best practices for using Dependency Injection?
Best practices include:
Avoid Over-Injection: Refactor classes with too many dependencies.
Prefer Constructor Injection: Makes dependencies explicit and code easier to maintain.
Design for Interface: Depend on abstractions (interfaces) rather than concrete implementations.
Dispose Services Properly: Ensure services implementing IDisposable are disposed of by the DI container.
11. How does Dependency Injection improve testability?
DI improves testability by allowing developers to inject mock dependencies into classes during unit testing, making it easier to isolate and test individual components.
12. What is the Inversion of Control (IoC) principle in Dependency Injection?
Inversion of Control (IoC) is a principle where the control of creating and managing dependencies is transferred from the class to an external component or framework, enhancing decoupling and modularity.
By addressing these frequently asked questions, developers can gain a deeper understanding of Dependency Injection and its practical applications in ASP.NET Core, leading to more efficient and maintainable software development.
//5.How are services injected in ASP.NET Core?
public class MyController : Controller
{
private readonly IMyDependency _myDependency;
public MyController(IMyDependency myDependency)
{
_myDependency = myDependency;
}
public IActionResult Index()
{
_myDependency.DoWork();
return View();
}
}
//8. How can services be registered conditionally in ASP.NET Core?
if (someCondition)
{
builder.Services.AddSingleton<IService, ServiceImplementationA>();
}
else
{
builder.Services.AddSingleton<IService, ServiceImplementationB>();
}
//9. How are scoped services injected into middleware in ASP.NET Core?
public async Task InvokeAsync(HttpContext context, IMyScopedService scopedService)
{
scopedService.DoWork();
await _next(context);
}
Results
#1. What is Dependency Injection?
#2. Which file is typically used to register services in ASP.NET Core? (ASP.NET Core 6 and after)
#3. Which service lifetime is suitable for services that maintain global state?
#4. What is one of the main benefits of Dependency Injection?
#5. How can scoped services be injected into middleware in ASP.NET Core?
Referal:
https://learn.microsoft.com/en-us/dotnet/core/extensions/dependency-injection